Two Blocks
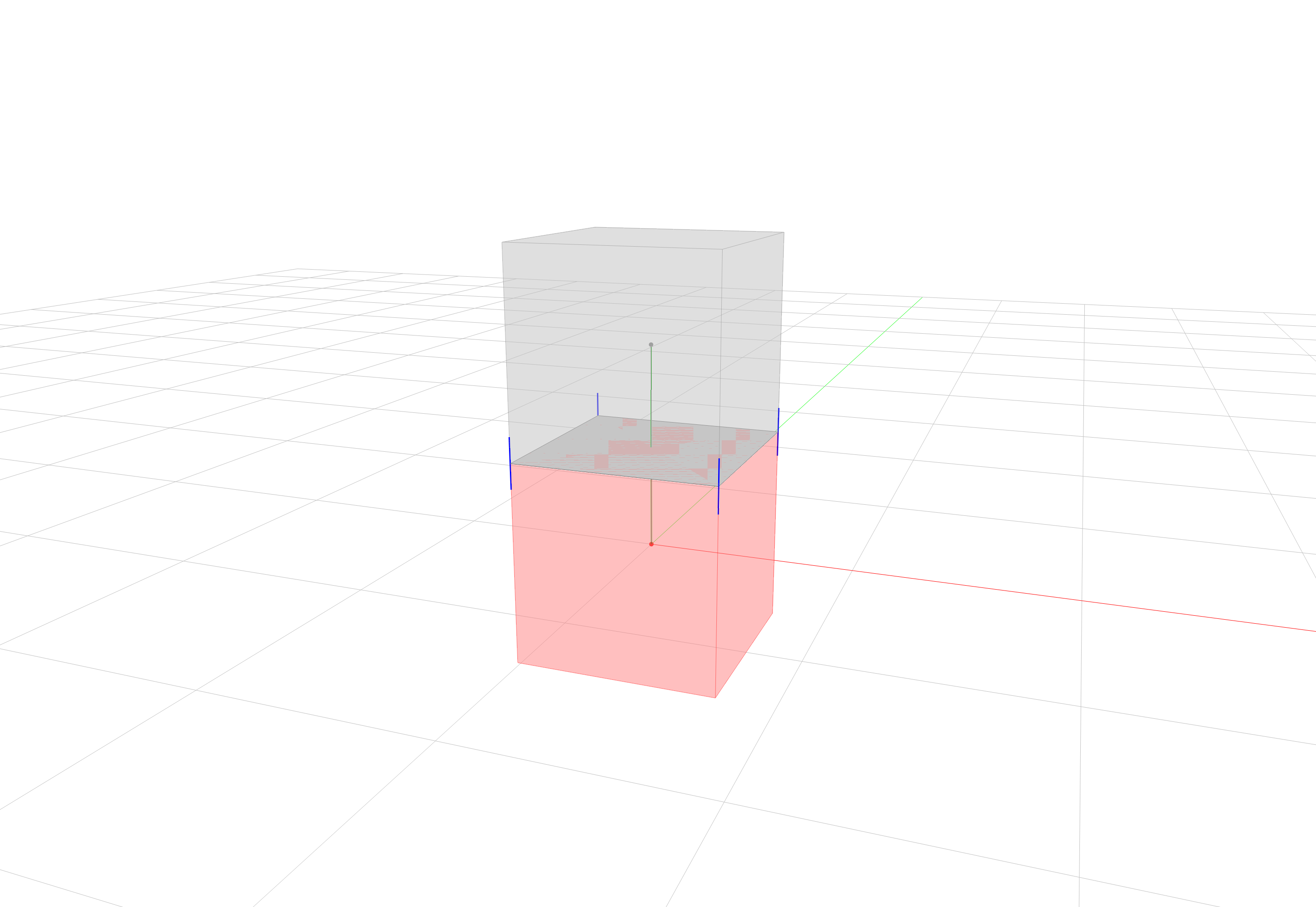
Summary
First, we construct an assembly from two simple blocks, one on top of the other. Then, we identify the interfaces of the assembly, and compute the contact forces between the blocks that result in static equilibrium with gravitational loads. Finally, we export the assembly to a JSON file and visualize the result with the DEM Viewer.
Equilibrium
The equilibrium is simple. The forces are equally distributed over the four corners of the interface, and the resultant is throught center. There is no friction.
Note
Note that this example uses compas_cra
for the equilibrium calculations.
If you don’t have compas_cra
installed,
or simply don’t want to compute the contact forces,
just comment out lines 3 and 48.
Code
import pathlib
from compas_cra.equilibrium import cra_solve
import compas
from compas.geometry import Box
from compas.geometry import Translation
from compas_assembly.algorithms import assembly_interfaces
from compas_assembly.datastructures import Assembly
from compas_assembly.datastructures import Block
from compas_assembly.viewer import DEMViewer
ASSEMBLY = pathlib.Path(__file__).parent / "two-blocks_assembly.json"
# =============================================================================
# Geometry
# =============================================================================
base = Box(1, 1, 1)
box1 = base.copy()
box2 = base.transformed(Translation.from_vector([0, 0, base.zsize]))
# =============================================================================
# Assembly
# =============================================================================
assembly = Assembly()
assembly.add_block(Block.from_shape(box1), node=0)
assembly.add_block(Block.from_shape(box2), node=1)
# =============================================================================
# Interfaces
# =============================================================================
assembly_interfaces(assembly, nmax=10, amin=1e-2, tmax=1e-2)
# =============================================================================
# Boundary conditions
# =============================================================================
assembly.set_boundary_condition(0)
# =============================================================================
# Equilibrium
# =============================================================================
cra_solve(assembly)
# =============================================================================
# Export
# =============================================================================
compas.json_dump(assembly, ASSEMBLY)
# =============================================================================
# Viz
# =============================================================================
viewer = DEMViewer()
viewer.view.camera.position = [0, -7, 1]
viewer.view.camera.look_at([0, 0, 0])
viewer.add_assembly(assembly)
viewer.run()