Shape
This tutorial provides a quick tour of the generation of Shape
.
The Shape can be created through a series of methods, such as:
from_meshes
: create the Shape by providing meshes representing the intrados and extrados.from_pointcloud
: create the Shape from pointclouds.from_library
: which creates a series of parametric Shapes that correspond to common masonry vaulted geometries.
The TNO library of Shapes is based on commom layouts which will be described herein.
Shapes Library
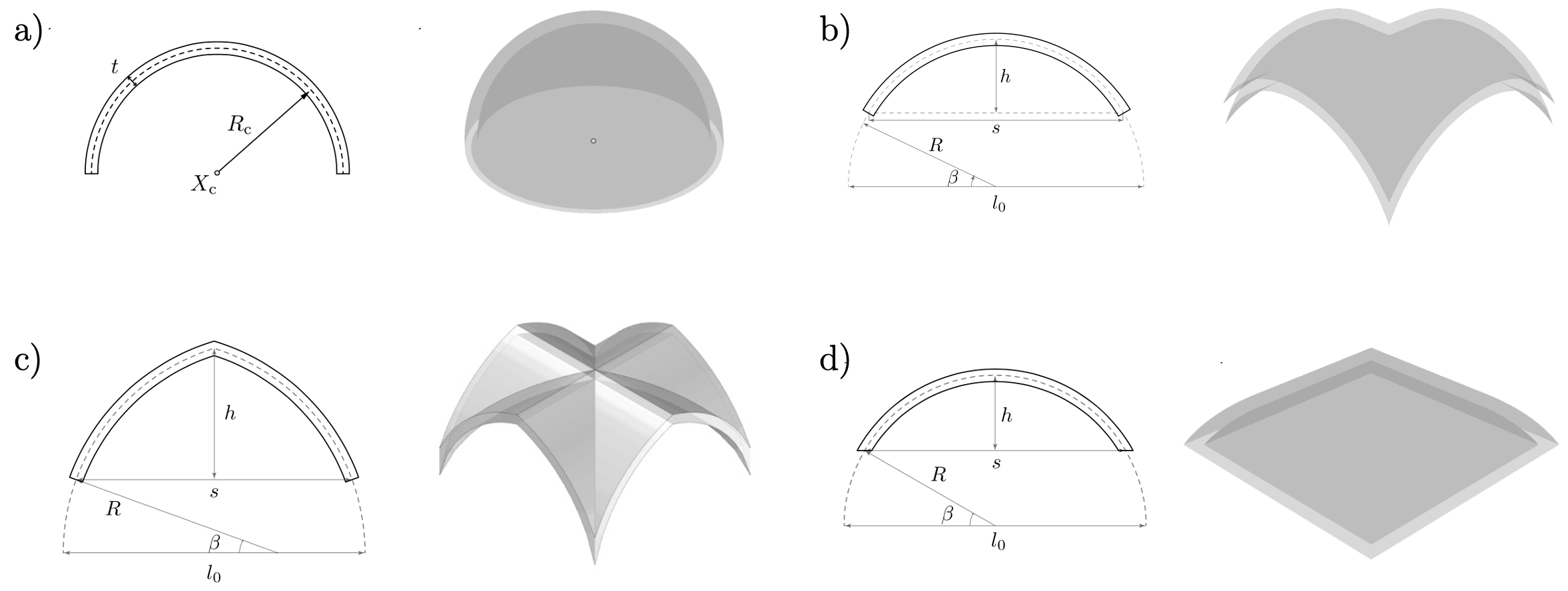
The methods to create each of these shapes is described herein.
create_dome
: hemispheric dome geometry defined from its radius, thickness
, center position
, etc. One example is presented below:
from compas_tno.shapes import Shape
shape = Shape.create_dome(center=[5.0, 5.0],
radius=5.0,
thk=0.5,
r_oculus=0.75)
create_crossvault
: rounded cross vault geometry defined from the span, thickness
, springing angle
, corner positions
. One example is presented below:
from compas_tno.shapes import Shape
shape = Shape.create_crossvault(xy_span=[[0.0, 10.0], [0.0, 10.0]],
thk=0.5,
spr_angle=30.0)
create_pointedcrossvault
: pointed cross vault geometry defined from the span, thickness
, springing angle
, corner positions
, height of center point
, and height of the boundary points
. One example is presented below:
from compas_tno.shapes import Shape
shape = Shape.create_pointedcrossvault(xy_span=[[0.0, 10.0], [0.0, 10.0]],
thk=0.5,
hc=7.0)
create_pavillionvault
: rounded pavillion vault geometry defined from the span, thickness
, springing angle
, corner positions
. One example is presented below:
from compas_tno.shapes import Shape
shape = Shape.create_pavillionvault(xy_span=[[0.0, 10.0], [0.0, 10.0]],
thk=0.5,
spr_angle=30.0)
To further explore the library of parametric shapes, check the Shapes
full documentation.
TNO Viewer
For 3D visualisation, the Viewer
can be used. This COMPAS View2 based visualisation enable to add multiple 3D objects in the scene, such as the shape, the thrust network, cracks, reaction forces, loads, etc. The viewer is used in the examples of this tutorial. The code to visualise the shape of a crossvault is:
from compas_tno.viewers import Viewer
from compas_tno.shapes import Shape
vault = Shape.create_crossvault(xy_span=[[0.0, 10.0], [0.0, 10.0]],
thk=0.5,
spr_angle=30.0)
view = Viewer(shape=vault)
view.draw_shape()
view.show()